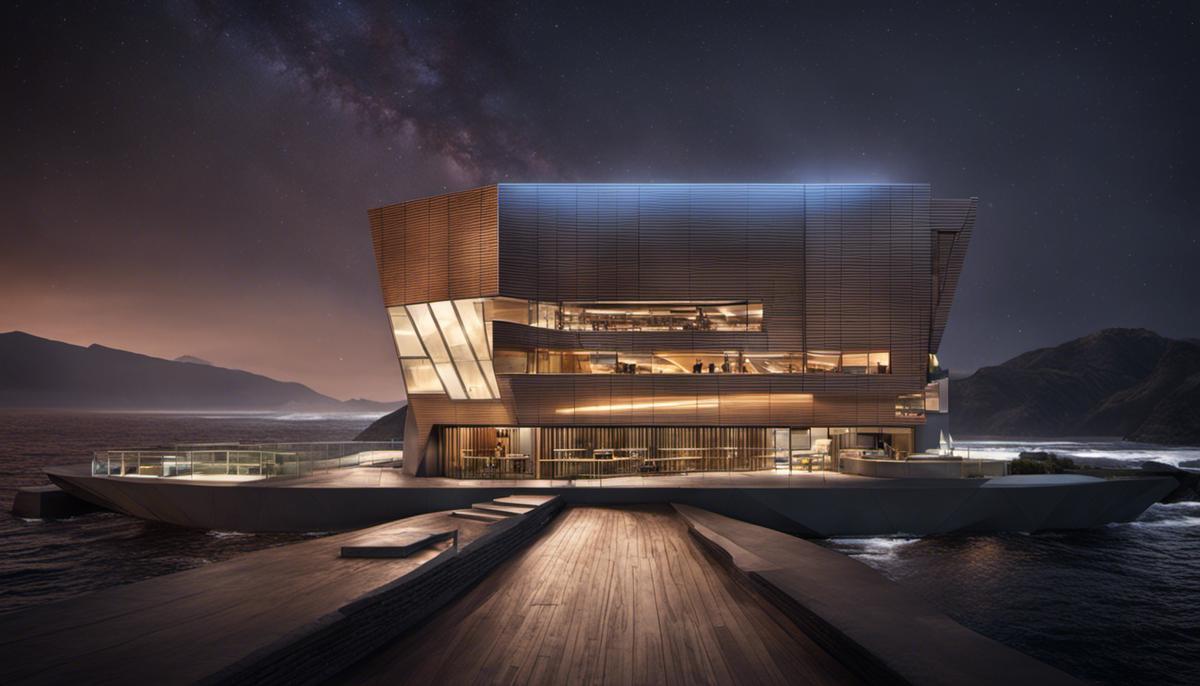
In an era where fast, robust, and scalable web applications are the norm, mastering the right tools can greatly amplify your development capabilities. Nuxt 3, with its potent mix of modular architecture, server-side rendering, and other core features, forms a quintessential part of any Vue.js application developer’s skillset. As enthusiasts and hobbyists stepping into this vast realm, understanding the architecture of Nuxt 3, imbibing the best practices for coding, learning how to manage data, and gaining insights into building and deploying Nuxt 3 applications effectively are the stepping stones to becoming proficient in Nuxt 3.
Understanding Nuxt 3 architecture
Understanding Nuxt 3 Architecture
Nuxt 3, the latest version of the Vue.js framework, is designed with a modular architecture. This architecture aids in making the codebase more maintainable and scalable. Each module in Nuxt 3 has its own clear functionality, which helps in breaking your application into discrete blocks of functionality that can be developed, tested, and debugged separately. This in turn increases the efficiency and speed of development.
Server-Side Rendering in Nuxt 3
One of the powerful features of Nuxt 3 is its ability to perform server-side rendering. It pre-renders Vue.js applications on the server before sending them to the client. This feature directly improves the performance of your application by rendering pages on the server-side before they reach the client’s browser, improving the user experience by drastically reducing load times.
Moreover, server-side rendering helps in Search Engine Optimization (SEO), as search engine crawlers can properly and efficiently index server-rendered applications, enhancing the visibility and discoverability of your website.
Core features of Nuxt 3
Nuxt 3 comes with several core features, making it a powerful tool for creating Vue.js applications. It’s equipped with a routing system that automatically generates Vue Router configuration based on your file tree of Vue files. This means you don’t have to manually set up routes; Nuxt will take care of it for you.
Another core feature is its robust Vue Meta management that makes managing your SEO easier. Your pages’ meta tags can be customized easily, from the default set defined in your configuration to each individual page.
Nuxt 3 also comes with a built-in store, and supports Vuex out of the box for managing your application’s state. The store is activated automatically when you create a store directory in your project.
Finally, Nuxt 3 has a plugin system which allows you to easily extend the framework’s core features. You can incorporate the Vue plugins, CSS libraries, and your custom plugins into your Nuxt 3 application seamlessly.
To properly work with Nuxt 3, one must familiarize oneself with these core features and understand how they work together under the hood to build a strong and efficient Vue.js application.
By having a good grasp of these features, you can enjoy the full capacity of the Nuxt 3 framework and streamline the creation of your Vue.js applications.

Best Practices for Coding in Nuxt 3
Clean Code Practices
Adhering to clean code practices will not only enhance your project’s readability and maintainability but also minimize the chance of introducing bugs and issues. Here are some best practices when using Nuxt 3:
- Stick to Single Responsibility Principle (SRP) — Each module, component, or function should only have a single responsibility. Avoid making your components or functions too big or multifaceted. Breaking down functionalities to smaller, reusable parts can greatly improve code readability and maintenance.
- Consistent Naming Conventions — Keep your naming conventions consistent. In Nuxt 3, it’s recommended to use kebab-case for components and camelCase for JavaScript variable names and function names.
- Comment Your Code — Comments can greatly improve the readability of your code, especially when working as part of a team. Strive to make your comments clear and concise, detailing what a specific block of code does.
- Error Handling — Always ensure that your application properly catches and handles errors. This can prevent unexpected behaviors and improve user experience.
Proper Utilization of Nuxt 3 Directory Structure
Understanding and utilizing the Nuxt 3 directory structure properly can greatly aid in the organization of your project. Here’s how to do it:
- Pages Directory — This is where you create your application’s routes based on the Vue.js components inside this directory. Keep your routes and associated pages organized and clearly named to make navigation within your codebase easier.
- Components Directory — All your Vue.js components go here. It’s automatically scanned by Nuxt 3 for components. Use subdirectories to categorize your components.
- Store Directory — If you’re using Vuex for state management, this directory is automatically transformed to Vuex Store.
- Middleware Directory — Any middleware you use in your application goes here. Middleware lets you define custom functions to run before rendering a page or a group of pages.
- Static Directory — Any static files that you want to directly accessible by your users, like robots.txt or sitemap.xml, should be placed in the static directory.
Ensuring Optimal Performance and SEO
Performance and SEO are critical aspects of web development. Below are some Nuxt 3 specific practices:
- Use the asyncData Method — This allows you to handle asynchronous operations before setting the component data. It can greatly improve the perceived loading time of your application.
- Disable Unused Features — Nuxt.js comes with many features out of the box. However, if your project does not utilize these features, make sure they’re disabled to reduce JavaScript payload.
- Optimize Images — Use lazy loading for images and use modern image formats such as WebP for better performance.
- Use Nuxt PWA Module — Transform your Nuxt.js app to a Progressive Web App (PWA) to provide an app-like experience to your users, offline support, and better SEO.
- Leverage Nuxt.js SEO Features — Nuxt.js provides a way to manage your website’s meta tags with the head method for better SEO. Use this feature to manage your app’s metadata.
By embracing these best practices, you can ensure your codebase remains manageable, your application performs optimally, and that it’s properly optimized for search engines with Nuxt 3.

Photo by krishna2803 on Unsplash
Handling Data with Nuxt 3
Understanding Nuxt 3 Data Handling Options
Nuxt 3 offers a range of options for handling data in your application. Among these options are new fetching methods and Vuex store, a prominent state management pattern and library useful in managing the state of an application.
Fetching Data in Nuxt 3
Nuxt 3 implements two fetching methods that you can use to get your data:
fetch()
: Fetching data on the server-side and hydrating it on the client-side.useFetch()
: Fetching data on the client-side using Composition API.
The fetch()
method can be placed at any component level and is called every time a component or page is loaded. It has access to Nuxt’s context object by using this
.
The useFetch()
method is a composable that can be used in the setup()
method to fetch data on the client side. Upon request, it returns an object with properties such as fetchState
, data
, error
, among others, which you can use to control the data fetching operation.
Remember that Nuxt 3’s fetch
methods offer an advantage over the regular JavaScript Fetch API or libraries like Axios as they are isomorphic, meaning they can be executed on both the client side and the server side.
Using Vuex Store in Nuxt 3
The Vuex store is a state management pattern and library which integrates deeply with Vue.js core to allow for efficient state management in large scale applications.
To create a Vuex store in Nuxt 3, you need to follow these steps:
- Make a directory named
store
in your project structure. - In the
store
directory, make aindex.js
file. This file will act as the root or main Vuex store file. - In the
index.js
file, you can define your root state, mutations, actions, and getters. - If your application requires more complex state management, you can create additional
*.js
files in thestore
directory. These files will be treated as namespaced Vuex modules.
While working with Vuex, remember that changes to state in Vuex can only be made through committing mutations. They are synchronous and keep track of changes in a way that is easy to understand and debug.
Also, if you find yourself needing to apply shared state logic across multiple components, consider using Vuex modules to avoid code repetition and promote reusable, maintainable code.
Consider this, using Vuex store and the new fetching methods in Nuxt 3 can help you create robust, scalable applications by efficiently managing application states and fetching data. You can choose the most suitable method based on your application requirements and design principles.

Building & Deploying Nuxt 3 Applications
Understanding Nuxt 3 App Types
Before jumping into building and deploying your Nuxt 3 applications, it’s crucial to understand the different types of applications that Nuxt 3 supports – Universal, Single-Page, and Static Generated.
- Universal applications, also known as Isomorphic or Server-Side Rendered (SSR), are the most common type where each page is generated by the server for each request.
- Single-Page applications, the entire application is loaded once and then never needs to go back to the server. Each new page is handled by Vue.js on the client-side.
- Static Generated applications, also known as Jamstack applications, are similar to universal apps, but the server-side rendering happens at build time.
Building Nuxt 3 Applications
To properly build your Nuxt 3 applications, follow the steps below:
- If you haven’t already, install the Nuxt 3 package by entering
npm i nuxt3 -g
in your command line. - Initialize your new project by running
npx nuxt3 init
in your preferred directory. - Once Nuxt 3 has been installed, you can run your applications locally using
npm run dev
. This step is crucial for testing and debugging your applications before deployment.
Deploying Nuxt 3 Applications
Once you’ve successfully built your Nuxt 3 application, the next step is to deploy it according to the type of application it is. Each type of Nuxt 3 application has different deployment procedures:
- Universal applications: Can be deployed to any environment capable of running Node.js, such as Heroku, AWS, or Google Cloud. It requires a server to handle HTTP requests and render the application’s pages.
- Single-Page applications: Can be uploaded directly to a static file server, a CDN, or a service like Netlify or GitHub Pages, as these applications don’t need a server to render pages.
- Static Generated applications: Can also be uploaded to any static file server or CDN. After you build your static site with
nuxt generate
, you’ll get adist
folder you can upload anywhere.
Remember, deploying your applications isn’t the last step. To ensure smooth functionality, regular updates and monitoring are needed. Tools such as Docker or Kubernetes can be helpful in managing your deployments.
Best Practices in Nuxt 3
Notwithstanding the differences in building and deploying these three types of applications, several common best practices can be adhered to:
- Always conduct extensive testing before deployment.
- Use middleware to control the rendering process.
- Keep your Nuxt application updated to the latest version.
- Regularly review and adhere to Nuxt’s performance checklist.
- Avoid placing large scripts in the initial payload, and use dynamic imports when possible.
- Consider using automated CI/CD pipelines for seamless deployment.

Photo by bostonpubliclibrary on Unsplash
With the knowledge of Nuxt 3’s modular architecture and familiarization with its core features, clean coding practices paired with an understanding of the directory structure, we are already on the journey to creating efficient applications. Managing data effectively using new fetching methods and Vuex store, and being able to build, deploy and choose the correct application type based on the need, completes our toolbox. As we continuously apply and hone these skills and practices, we robustly stride forward in our journey of mastering Nuxt 3, thereby promising an enhanced web development experience with every new application we develop.
Let Writio’s AI brilliance produce exceptional content for your website! This article was crafted by Writio.