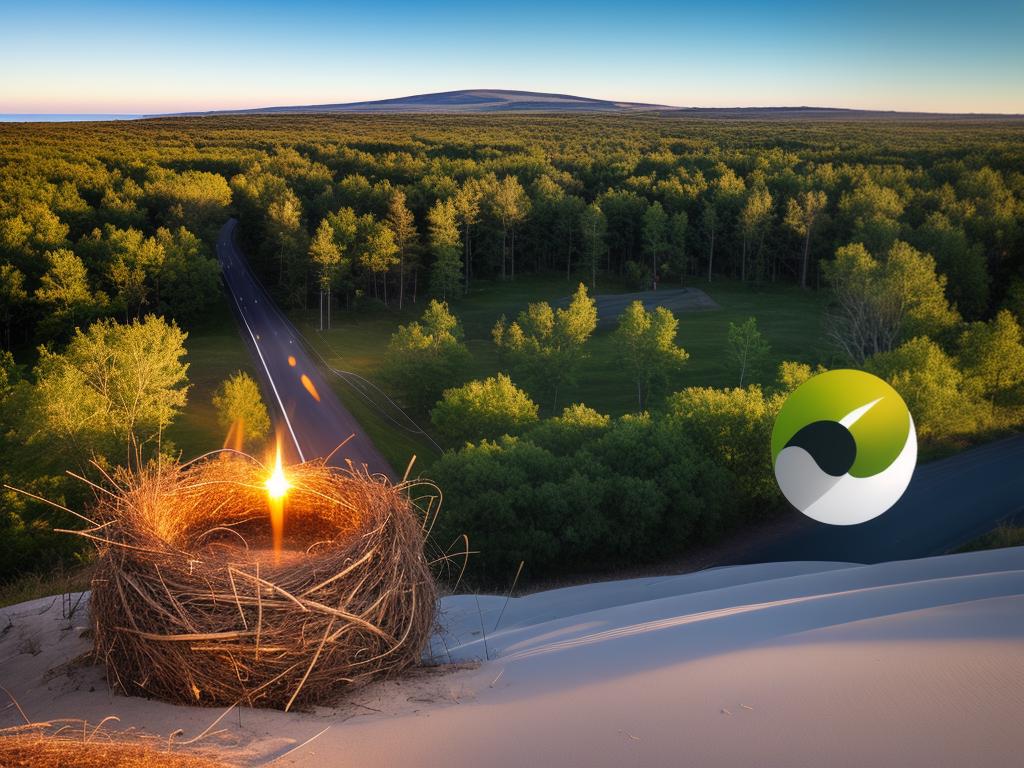
With an increasing shift toward the development of robust, scalable, and maintainable server-side applications, Nest Js has emerged as a breakout star in the sphere of web development. Embracing the power of TypeScript and drawing from the paradigms of Angular, Nest Js offers a remarkable foundation to craft server-side applications with ease and precision. In the odyssey of learning to create a Nest Js API, this essay will help illuminate your path, armed with a knowledge of its core foundations, principles, and implementing techniques. Beginning with a conceptual understanding of Nest Js, you will venture into learning about its modules and controllers, appreciate the role of services and providers, and delve into the nuances of error handling and validation.
Introduction to Nest Js
Introduction to Nest Js
Nest Js is a progressive Node.js framework for building efficient and scalable server-side applications by using JavaScript. Nest Js provides an out-of-the-box application architecture that allows developers to create highly testable, scalable, loosely coupled, and maintainable applications.
Nest Js combines elements of Object Oriented Programming (OOP), Functional Programming (FP), and Functional Reactive Programming (FRP). Moreover, it employs TypeScript, but still preserves compatibility with pure JavaScript.
Benefits of Nest Js
- It uses TypeScript for development which improves code quality and understandability.
- It has a modular structure that organizes the code into separate modules based on features or functionality.
- It is highly scalable due to its modular architecture.
- Nest Js provides detailed documentation that makes it easier for beginner developers to learn and understand.
- It supports microservice architecture which allows developers to build scalable and complex backend applications.
Applications of Nest Js
- Real-time applications like Chat systems or GPS tracking systems
- E-commerce applications which require complex operations and components
- Enterprise web applications
Setting Up Node and Nest Js
Before you start building with Nest Js, ensure you have Node.js and npm (Node Package Manager) installed on your computer.
- To check if you have Node.js installed, run this command in your terminal:
- To check if you have npm installed, run this command:
- After setting up Node.js and npm, you can install Nest.js by running:
node -v
If Node.js is installed, it will display the version, otherwise, it will prompt you to install it.
npm -v
Similar to Node.js, it will display the version or prompt you to install it.
npm i -g @nestjs/cli
This will install the Nest.js CLI globally on your machine.
Creating Your First Nest Js Application
Now, you’re ready to create your first Nest Js application. Use the following steps:
- To generate a new project, use the following command:
- This command will prompt you to choose a package manager, select either npm or Yarn.
- The installation process will create a new directory with your project name and generate a Nest.js project structure inside it.
- Navigate into the project directory:
- To start the server, run:
- Open your preferred web browser and navigate to http://localhost:3000. You should see a “Hello World” message, indicating that your Nest Js application is working.
nest new project-name
Replace “project-name” with your preferred project name.
cd project-name
npm run start
Remember, Nest Js provides a modular structure – allowing you to neatly arrange and organise your code, so take advantage of it and start building!

Modules and Controllers
Introduction to Nest Js
Nest Js is a versatile framework built using Node.js. It leverages the flexibility and power of JavaScript as a backend language. Nest Js incorporates Object-Oriented Programming (OOP), Functional Programming (FP), and Functional Reactive Programming (FRP) paradigms to provide developers with an all-encompassing tool for creating scalable and maintainable server-side applications.
Understanding Modules
A module plays a pivotal role in structuring Nest Js applications. It’s a class annotated with @Module()
decorator. The decorator provides metadata that Nest Js uses to organize the application structure.
Module Implementation
Each Nest Js application has at least one module, the ‘root’ module. You can identify it with the @Module()
decorator. The decorator contains objects that hold relevant metadata:
- providers: These are the providers to be available within the scope of the module.
- controllers: These are the set of controllers defined within the module.
- imports: This lists the imported modules that export the providers which are needed in this module.
- exports: These are the subset of providers that are provided by this module and can be used by other modules.
To create a module, you can use the Nest CLI command: nest g module module_name
.
Understanding Controllers
Controllers are responsible for controlling the flow of data between the end user and the application. They handle incoming requests and return responses to the client.
Controller Implementation
A controller is a class decorated with @Controller
decorator. The decorator can take an optional string argument to specify the path that the controller will handle.
For instance, if you define @Controller('tasks')
, it means that this controller will work with any endpoint that starts with ‘/tasks’.
Controllers can then have handler functions that respond to specific HTTP methods (GET, POST, PUT, DELETE, etc.). These methods are decorated with corresponding method decorators such as @Get()
, @Post()
, @Put()
, @Delete()
etc.
To create a controller, use the Nest CLI command: nest g controller controller_name
.
Using Modules and Controllers to Develop a Nest Js API
With an understanding of Modules and Controllers, you can now use them to start building your API. You start by declaring your controllers and providers in the module, then handle routing and data manipulation within the controllers. The modularity of Nest Js allows you to separate concerns and keep your code organized, making it easier for developers to understand and maintain the codebase.
In conclusion, modules and controllers play major roles in organizing and structuring your application in Nest Js. Over time, utilizing these components effectively can lead you to develop clean, maintainable, and scalable API’s.

Services and Providers
Understanding Services and Providers
Services and providers in Nest Js are integral to developing APIs. They help manage the logic of your applications and offer efficient ways to achieve modularity and injectability. In essence, services are classes that are marked with the @Injectable() decorator and can be used anywhere within your app, while providers are a more general term that Nest Js uses to include services, repositories, factories, values, and custom-provided logic.
Creating a Service
To create a Nest Js service, follow these steps:
- First, use the CLI command
nest g service serviceName
where ‘serviceName’ is the name of your new service. This will create a new service file in a folder with the same name, and update the module where the service has been generated. - The newly created service will be a class decorated with
@Injectable()
and should look like this:
import { Injectable } from '@nestjs/common';
@Injectable()
export class ServiceNameService {}
Utilizing a Service
To utilize a service, you need to inject it into the desired module. Here’s how to do it:
- Import the service into the component where you want to use it.
- Inject it into the component’s constructor.
import { ServiceNameService } from './serviceName.service';
constructor(private serviceNameService: ServiceNameService) {}
Once injected, you can use the service’s methods through this.serviceNameService.methodName()
.
Creating a Provider
Providers can be created by using a plain value, factory, class, and asynchronous factory. Here’s an example of creating a class provider:
- First, define a class.
export class CatsService {
constructor(private connection: Connection) {}
}
- Provide it in the module where you’d like to use it.
@Module({
providers: [
CatsService,
{
provide: Connection,
useValue: databaseConnection,
},
],
})
export class CatsModule {}
- You can then inject the provider in your classes.
constructor(private catsService: CatsService) {}
Providers are somewhat similar to services but offer a more generalized concept and flexible usage when developing APIs in Nest Js. Services are essentially one type of provider that have the extra advantage of being injectable throughout the entire application. They manage your application logic and are marked with the @Injectable()
decorator.
To summarize, services are a way of encapsulating logic that isn’t tied to any specific object but can be used application-wide, and providers offer a way to use this logic in a way that is flexible and fits the concept you’re working within.

Error Handling and Validation
Introduction to Error Handling and Validation in Nest Js
Handling errors and validating requests in Nest Js is fundamental for building a robust and secure API. With proper error handling, your application can anticipate and respond to issues that may occur during execution. Similarly, validation ensures that the data your application receives is accurate and in the expected format.
Nest Js Error Handling
At the heart of error handling in Nest Js is the built-in exceptions layer, which is responsible for processing all unhandled exceptions across your application.
- Throwing standard exceptions: The simplest way to handle errors is by using built-in HTTP exceptions. Here’s how you can throw standard exceptions:
- Creating custom exceptions: Nest Js allows you to create custom exceptions if the built-in exceptions don’t meet your requirements. Create a class that inherits from the base HttpException class:
throw new NotFoundException();
export class CustomException extends HttpException {
constructor() {
super('Custom Message', HttpStatus.I_AM_A_TEAPOT);
}
}
Then, you can throw your custom exception like a standard one:
throw new CustomException();
Validation in Nest Js
Nest Js utilizes the powerful class-validator package for handling input validation. Here’s a basic example of how to validate input data:
- Install the necessary dependencies:
- Create a DTO (Data Transfer Objects) and apply validation decorators:
- Use the ValidationPipe: To leverage the validation rules defined in the DTO, use ValidationPipe:
npm i class-validator class-transformer
import { IsEmail, IsNotEmpty } from 'class-validator';
export class CreateUserDto {
@IsEmail()
email: string;
@IsNotEmpty()
password: string;
}
This indicates that the email should be a valid email address and the password should not be empty.
import { Body, Controller, Post, ValidationPipe } from '@nestjs/common';
import { CreateUserDto } from './dto/create-user.dto';
@Controller('users')
export class UsersController {
@Post()
create(@Body(ValidationPipe) createUserDto: CreateUserDto) {
// ...
}
}
Guarding Routes
Guards in Nest Js allow controlling whether a request can proceed to the route handler. Here’s a simple example:
- Creating a Guard: Create a guard using the @nestjs/common and @nestjs/passport packages:
- Using the Guard: Use the guard by attaching an @UseGuards() decorator to the route handler:
import { Injectable, UnauthorizedException } from '@nestjs/common';
import { AuthGuard } from '@nestjs/passport';
@Injectable()
export class JwtAuthGuard extends AuthGuard('jwt') {
handleRequest(err, user, info) {
// Handle your error, user, and info instances here
if (err || !user) {
throw err || new UnauthorizedException();
}
return user;
}
}
@Controller('users')
export class UsersController {
@UseGuards(JwtAuthGuard)
@Get('me')
getProfile(@Request() req) {
return req.user;
}
}
This verifies that only authenticated users can access the “me” route.
With these tools at your disposal, you’ll be equipped to handle errors and validate data effectively in your Nest Js API. Experiment with these concepts and dig deeper into each one to further refine handling of errors and validation in your application.

As you look back on this quest into the world of Nest Js, you have journeyed from the foundational knowledge, through the crux of its features and functionalities, and right into the heart of handling errors and validation. Now, you are equipped with the right set of tools, not only to develop a Nest Js API but also to further experiment, learn and grow with the framework. The understanding of Nest Js’s modules, controllers, services, and providers now forms a part of your web development arsenal, allowing you to program with clarity and efficiency, thereby enhancing your ability to develop rich internet applications with greater ease. As you forge ahead in your web development journey, remember the lessons learned and apply them with creativity and passion to build truly remarkable applications.