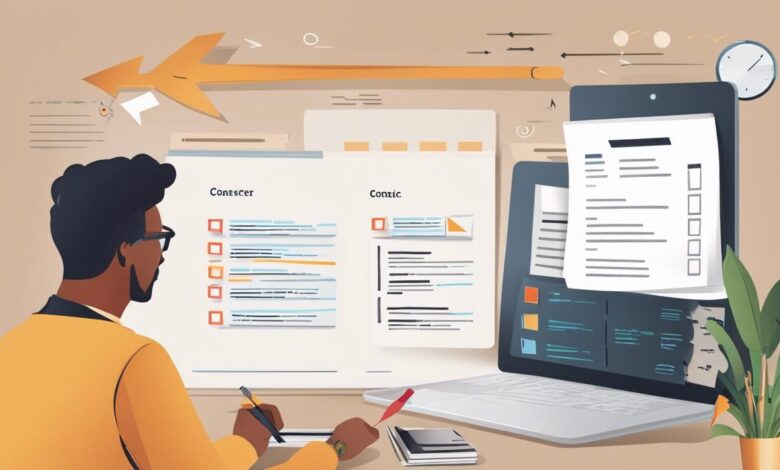
Embarking on the journey to master Python is an adventure teeming with opportunities to streamline code and enhance efficiency. Among the most powerful tools in the Pythonic toolbox are List Comprehensions, Lambda Functions, and Generator Expressions. These features not only epitomize the elegance and simplicity for which Python is renowned but also serve to heighten one’s programming acumen. This essay delves deep into the nuances of these constructs, unveiling the art of crafting succinct and performant code. Through a comprehensive exploration of these topics, enthusiasts and hobbyists alike will discover how to harness the capabilities of Python to the fullest, transforming complex tasks into lines of code that are both beautiful and functional.
List Comprehensions
The Elegance of List Comprehensions in Python Programming
In the realm of Python programming, the efficiency of code is not only measured by its execution speed but also by its readability and conciseness. Among the pantheon of techniques available to the Python developer, list comprehensions offer a sterling method for transforming and condensing complex loops and operations into a single, readable line of code. This discussion will illuminate the mechanics and applications of list comprehensions, fostering an understanding of how they can streamline Python code.
List comprehensions provide a succinct and intuitive approach to generating lists. Born from the functional programming paradigm, their incorporation into Python underscores the language’s commitment to code clarity and elegance. Essentially, list comprehensions allow for the construction of new lists by succinctly iterating over sequences, applying an expression to each element.
To begin utilizing list comprehensions, one must understand their basic structure, which can be described as follows:
[expression for item in iterable if condition]
This structure encapsulates a loop and a conditional statement (which is optional) into a single line, where ‘expression’ is any Python expression, ‘item’ represents the individual elements that are drawn from the ‘iterable’, and ‘condition’ is a predicate that filters elements.
Consider a common scenario where one might wish to create a list of squared numbers. In lieu of the verbose and potentially labyrinthine traditional for loop, observe the economy of expression in the list comprehension:
squared_numbers = [x**2 for x in range(10)]
The alternative, an expanded loop, may appear as such:
squared_numbers = []for x in range(10):
squared_numbers.append(x**2)
Clearly, the comprehension not only reduces the volume of code but enhances readability by encapsulating intent. By employing this construct, developers remove extraneous scaffolding, allowing the essential logic to prevail.
Comprehensions also confer an avenue to implement filtering. Introducing a conditional expression, list comprehensions can selectively include elements in the resulting list:
even_squares = [x**2 for x in range(10) if x % 2 == 0]
Here, only the squares of even numbers are compiled, illustrating the powerful synthesis of iteration and conditionals.
Performance-wise, list comprehensions can have a favorable impact on runtime, provided the expressions and conditions are not exceedingly complex. It is imperative to recognize that list comprehensions are not a panacea and should be deployed judiciously. Notably, overly intricate comprehensions can impede comprehensibility, thus betraying the Pythonic ethos of code legibility.
In sum, list comprehensions embolden the Python developer to write more concise and efficient code, elegantly weaving together iterations and conditional logic into a coherent and readable tapequetry. Integrating this feature judiciously within Python programs aligns with the laudable pursuit of code brevity without negating readability. Engaging with list comprehensions not solely as a tool for code compression, but as a means to encapsulate and purify logical constructs is a noble endeavor in the art of programming.

Lambda Functions
Lambda functions in Python
Otherwise known as anonymous functions, lambda functions are a concise mechanism to represent small, one-off functions that need not be explicitly named. Much like list comprehensions, lambda functions contribute to the elegance and readability of the Python programming language, although their application warrants careful consideration to maintain code clarity.
A lambda function is composed of a single expression statement and is typically used when a function is to be utilized for a short duration, without the tedium of formally defining a function using the def
keyword. The syntax of the lambda function is straightforward; it begins with the lambda keyword, followed by a list of parameters, a colon, and finally, the expression it encapsulates. For example, a lambda to square a number might be written as: lambda x: x ** 2
.
In terms of practical application, lambda functions integrate smoothly with higher-order functions that accept other functions as arguments. A prime example of these are the built-in functions map()
, filter()
, and reduce()
. With map()
, a lambda function can transform each item in a list, much like list comprehensions do, but with potentially improved readability for very simple transformations. For instance, raising a list of integers to the power of two can be accomplished by map(lambda x: x ** 2, integer_list)
. The filter()
function can be accentuated with lambda expressions to exhibit precise control over which elements to include in a resulting list, such as filter(lambda x: x > 0, number_list)
to extract positive numbers.
Lambda functions also flourish within the paradigms of event-driven programming and callbacks, providing a convenient way to pass a small piece of executable functionality without extraneous function definition overhead.
Despite the advantages of succinctness, lambda functions should be used judiciously. They can introduce obscurity and impede readability if the expression becomes too complex or if overused. The single-expression limitation ensures that lambda functions remain simple; however, if the logic of the expression becomes involved, a named function is preferable for the sake of transparency and ease of testing.
Furthermore, it is not unusual for lambda functions to be less intuitive for beginners. Their concise nature, while powerful, can also lead to dense lines of code that may challenge less experienced developers. Hence, they are most advantageous when they improve the clarity and brevity of a code snippet and are understandable at a glance.
To maintain the highest standards of code maintainability, lambda functions should be employed when a simple, temporary function is necessary, and their introduction into the codebase enhances readability and function. Restraint is recommended so as not to sacrifice the self-documenting feature of Python, a cornerstone of its widespread adoption and the clarity for which it is renowned.

Generator Expressions
In the domain of Python programming, memory efficiency is a paramount consideration as it influences not only the execution speed of a program but also its scalability and resource consumption. In light of these concerns, generator expressions emerge as a sophisticated tool that enables programmers to iterate over data streams with high memory efficiency.
Generator expressions are closely related to list comprehensions; however, unlike list comprehensions that construct a complete list in memory, a generator expression returns a generator object. This object adheres to the iterator protocol and generates items on-the-fly rather than storing them in memory all at once. It is defined using a similar syntactic structure to list comprehensions but with parentheses instead of square brackets.
Consider an operation where one intends to process items within a substantial dataset, performing computations or transformations on each item. Utilizing list comprehensions or storing an entire list in memory could be wasteful and impractical for large datasets. Instead, generator expressions provide a potent alternative by computing items as they are needed for consumption, thus, significantly reducing the memory footprint of such an operation.
Furthermore, when incorporating generator expressions in one’s code, it is possible to achieve a "pipeline" of operations. Here, multiple generator expressions can be linked together, with each generator consuming and yielding items to the next in sequence. This chaining of generators is advantageous as it permits the creation of complex data processing streams without the compounding memory cost associated with intermediate data structures.
This principle of on-demand generation is central to understanding the implication of generator expressions on memory efficiency. During the iteration process, a generator expression fulfills requests for values one at a time, relinquishing control after each yield and resuming where it left off for the next request. This characteristic enables handling streams of data that would be prohibitively large to hold in memory at once, such as processing records from extensive databases or reading from massive files.
An essential aspect of using generator expressions is their compatibility with built-in Python functions and constructs that consume iterators, such as sum()
, min()
, max()
, and loop constructs. These functions can operate on generator expressions directly without necessitating a full list, thus perpetuating the memory efficiency intrinsic to generators.
In conclusion, understanding and utilizing generator expressions is indispensable for Python programmers who seek to optimize the memory efficiency of their code. Generators enable the processing of large or infinite data streams with minimal memory usage, thereby facilitating efficient and scalable software development. It is incumbent upon practitioners in this field to integrate generator expressions judiciously and leverage their distinct advantages in the appropriate context to achieve both operational efficiency and maintain code readability.

Having traversed the realms of Python’s List Comprehensions, Lambda Functions, and Generator Expressions, we emerge with a refined understanding of how these features can dramatically elevate the caliber of our coding practices. As hobbyists and enthusiasts, our foray into these topics is more than an academic exercise—it is a practical toolset that prepares us to tackle real-world problems with greater proficiency and creativity. Let this be a stepping stone to greater explorations in Python, fostering a style of coding that is as efficient as it is eloquent, ensuring that each script we write bears the hallmark of our deepened insight and newfound skills.
Enhance your website with Writio, the ultimate AI content writer. Discover high-quality articles crafted by Writio, your reliable partner in content creation.